Make social login using Laravel in 5 minutes for rich UX.
Social login is a powerful feature that can enhance your web application’s user experience. It allows users to log in using their Google, Facebook, or other social media accounts, providing a faster and more secure authentication process. In this guide, we’ll walk you through how to implement social login using Laravel and the Socialite package.
We’ll use Google and Facebook as examples, but once you understand the basics, you’ll be able to add other social login providers easily.
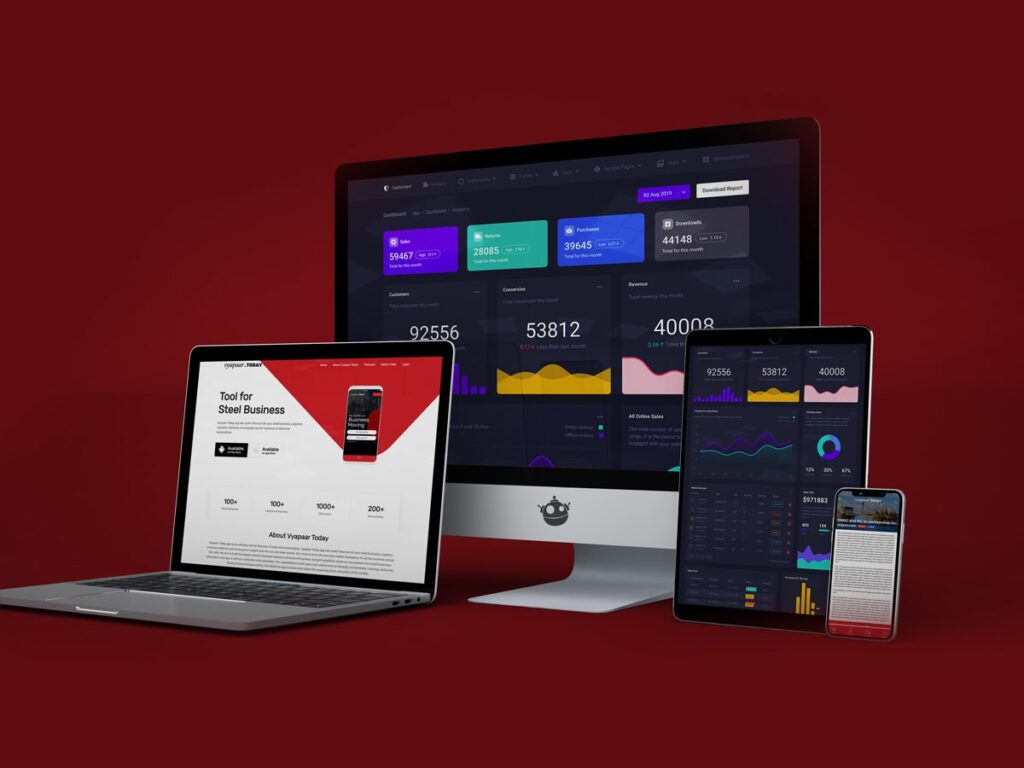
1. Run the Project
Before we begin, make sure you have a Laravel project set up and running. If you don’t have one, you can quickly create a new project using Composer.
Command:
composer create-project --prefer-dist laravel/laravel social-login-app
cd social-login-app
php artisan serve
This command will create a new Laravel project and start the development server. Once it’s running, you can proceed with the integration of social logins.
2. Get the Socialite Package
To integrate social logins in Laravel, we’ll use the Socialite package. Socialite makes it easy to authenticate with OAuth providers such as Google, Facebook, GitHub, and others.
Install Socialite:
composer require laravel/socialite
Once the package is installed, open the config/app.php
file and add the Socialite service provider to the providers
array if it’s not automatically added.
Example:
'providers' => [
// Other Service Providers
Laravel\Socialite\SocialiteServiceProvider::class,
],
Also, add the alias in the aliases
array:
'aliases' => [
// Other Facades
'Socialite' => Laravel\Socialite\Facades\Socialite::class,
],
3. Add Config
Next, we need to configure the services for Google and Facebook. Open the config/services.php
file and add the following configuration:
'google' => [
'client_id' => env('GOOGLE_CLIENT_ID'),
'client_secret' => env('GOOGLE_CLIENT_SECRET'),
'redirect' => env('GOOGLE_REDIRECT_URI'),
],
'facebook' => [
'client_id' => env('FACEBOOK_CLIENT_ID'),
'client_secret' => env('FACEBOOK_CLIENT_SECRET'),
'redirect' => env('FACEBOOK_REDIRECT_URI'),
],
This tells Laravel where to find the credentials for Google and Facebook OAuth.
4. Update Routes
Next, we’ll update the routes to handle social logins. Open the routes/web.php
file and add the following routes:
use Laravel\Socialite\Facades\Socialite;
Route::get('/login/{provider}', [App\Http\Controllers\Auth\LoginController::class, 'redirectToProvider']);
Route::get('/login/{provider}/callback', [App\Http\Controllers\Auth\LoginController::class, 'handleProviderCallback']);
These routes will handle the redirection to the OAuth provider and the callback after authentication.
5. Create OAuth App
For social login to work, you need to create OAuth apps on both Google and Facebook. You will get the required client_id
, client_secret
, and redirect_uri
for each platform.
- Google: Go to Google Developer Console, create a new project, and set up OAuth credentials for Web Applications. Provide the callback URL as
http://your-domain.com/login/google/callback
. - Facebook: Go to Facebook Developer Portal, create a new app, and configure the OAuth credentials under “Settings > Basic”. Set the callback URL as
http://your-domain.com/login/facebook/callback
.
Once created, note down the client_id
, client_secret
, and redirect_uri
for both providers.
6. Update Environment Variables
Add the credentials you obtained into the .env
file of your Laravel project.
Example:
GOOGLE_CLIENT_ID=your-google-client-id
GOOGLE_CLIENT_SECRET=your-google-client-secret
GOOGLE_REDIRECT_URI=http://your-domain.com/login/google/callback
FACEBOOK_CLIENT_ID=your-facebook-client-id
FACEBOOK_CLIENT_SECRET=your-facebook-client-secret
FACEBOOK_REDIRECT_URI=http://your-domain.com/login/facebook/callback
This ensures that the application connects to the correct OAuth providers using your credentials.
7. Check Application
Once the setup is complete, you can now test your social login. Visit the login route for Google and Facebook respectively:
http://your-domain.com/login/google
http://your-domain.com/login/facebook
If everything is set up correctly, you should be redirected to the respective social login provider and then back to your application after a successful login.
In your LoginController
, you will need to handle the redirection and user data:
public function redirectToProvider($provider)
{
return Socialite::driver($provider)->redirect();
}
public function handleProviderCallback($provider)
{
$user = Socialite::driver($provider)->user();
// Here, you can retrieve user info and log the user in or register them
// Example:
// $user->getName(), $user->getEmail(), $user->getAvatar()
// Add logic to store user in DB or authenticate user
}
8. Best Practices
- Security: Always validate the OAuth responses properly to ensure the data comes from a trusted source.
- Error Handling: Ensure you handle any exceptions that may occur during the authentication process (e.g., invalid tokens or expired sessions).
- User Experience: Customize the user flow for the best experience. Offer users multiple social login options and fallback mechanisms if one fails.
- Keep Dependencies Updated: Socialite and its providers may release updates or security patches. Keep your dependencies updated to avoid vulnerabilities.
Conclusion
Adding social login to your Laravel project enhances user convenience and speeds up the onboarding process. With Socialite, Laravel makes it easy to implement secure and user-friendly social login. By following the steps outlined in this guide, you can integrate both Google and Facebook login with minimal effort and give your users a smooth, modern authentication experience.
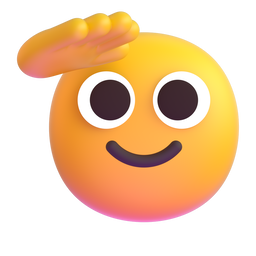
Ready to implement or upgrade existing CRM solution?
Get in touch with our expert to create personalized CRM or upgrade existing solution. Use SalesAnalytika platform to create unlimited leads, sync and integrate. Get free trial for one month for free to see if our CRM make any change!