Solving N+1 Problem in Laravel: Optimizing Database Queries for Performance
Introduction
The N+1 query problem is one of the most common performance bottlenecks in applications using ORMs (Object-Relational Mapping). In Laravel, this issue occurs when your application makes one query to fetch a dataset and then makes an additional query for each item in the dataset to retrieve related data. This results in N+1 queries, leading to increased database load and slower performance.
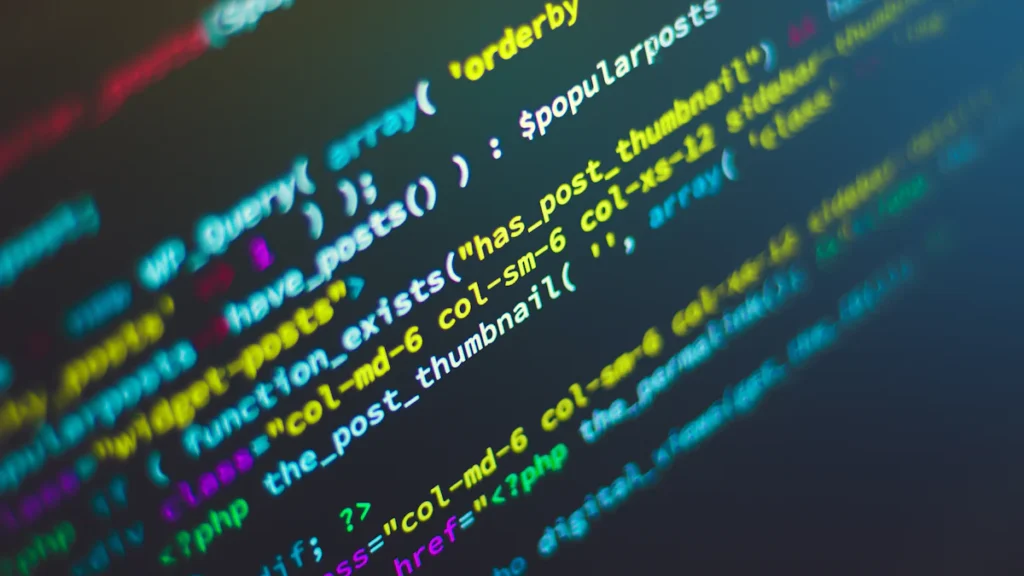
Why Does the N+1 Problem Occur?
In Laravel, the N+1 issue typically arises when loading related data across tables. If not handled properly, fetching relationships between models (e.g., users and their posts) can result in running multiple queries instead of a single optimized one.
For example, consider this scenario:
// Example: Fetch all users and their posts
$users = User::all(); // 1 query to get all users
foreach ($users as $user) {
$posts = $user->posts; // N additional queries to get each user's posts
}
In this case, for each user, Laravel will execute a separate query to retrieve their posts, leading to N+1 queries. If there are 100 users, you will end up executing 101 SQL queries (1 for fetching all users + 100 for fetching each user’s posts).
Why Does it Happen?
The N+1 problem typically arises when dealing with eager-loading relationships between tables, especially in ORM (Object-Relational Mapping) tools like Eloquent in Laravel. Since ORM abstracts SQL queries into object manipulation, developers sometimes miss optimizing how related data is fetched, leading to multiple queries instead of optimized, single query joins.
How N+1 Impacts Application Performance
The N+1 query problem can severely impact application performance, especially when dealing with large datasets. It causes:
- Database Overload: Multiple queries are sent to the database, significantly increasing the database load.
- Increased Latency: More queries mean more round trips to the database, increasing the response time of your application.
- Scalability Issues: As the data grows, the performance hit becomes even more pronounced, affecting the overall scalability and user experience of the application.
Solving the N+1 Problem in Laravel
Laravel provides Eager Loading to resolve the N+1 issue efficiently. Instead of fetching related data in separate queries, eager loading retrieves the related data in one optimized query using a JOIN
.
Here’s how to solve it:
1. Use Eager Loading (with
)
Instead of fetching the users and their posts separately, you can use the with()
method to load relationships in one go:
// Optimized query with eager loading
$users = User::with('posts')->get();
This will fetch all users and their related posts in a single query, solving the N+1 problem. The generated SQL query would look something like this:
SELECT * FROM users
LEFT JOIN posts ON posts.user_id = users.id;
2. Use load()
for Lazy Eager Loading
If you’ve already fetched your users but want to load related data later, you can use the load()
method:
$users = User::all();
$users->load('posts');
This is useful when you want to decide on fetching related data after the initial query execution, but still avoid the N+1 problem.
3. Eager Load Nested Relationships
If you need to load deeper relationships, you can chain eager loading for nested relationships:
// Eager loading posts and comments associated with users
$users = User::with(['posts', 'posts.comments'])->get();
4. Batch Queries for Optimized Fetching
Sometimes, a combination of eager loading and batching can help reduce queries and improve overall performance. Laravel allows you to write custom joins or use batching patterns for more complex data fetching scenarios.
Additional Techniques to Optimize
- Limit Data with
select()
Eager loading all columns can sometimes still be inefficient. You can limit the columns to only what’s needed:phpCopy code$users = User::with(['posts:id,title,user_id'])->select('id', 'name')->get();
- Avoid Deep Eager Loading
Loading too many nested relationships can still result in large, complex queries. Be mindful of how much data you’re eager loading, and use pagination when appropriate.
Conclusion
The N+1 problem can sneak into Laravel applications when dealing with related data across tables. It can lead to database overload, slow queries, and scalability issues. By using Laravel’s eager loading (with
, load
), you can optimize your queries, reduce database round trips, and improve your application’s performance.
Always remember:
- Use eager loading to solve the N+1 problem.
- Limit the data you select for better efficiency.
- Monitor your queries with Laravel Debugbar or Telescope to spot N+1 issues early.